The built-in “MareBackup” scheduled task is susceptible to a trivial executable search order hijacking, which can be abused by a low-privileged user to gain SYSTEM
privileges whenever a vulnerable folder is prepended to the system’s PATH
environment variable (instead of being appended).
Insomni’hack 2025 – GuLosity writeup
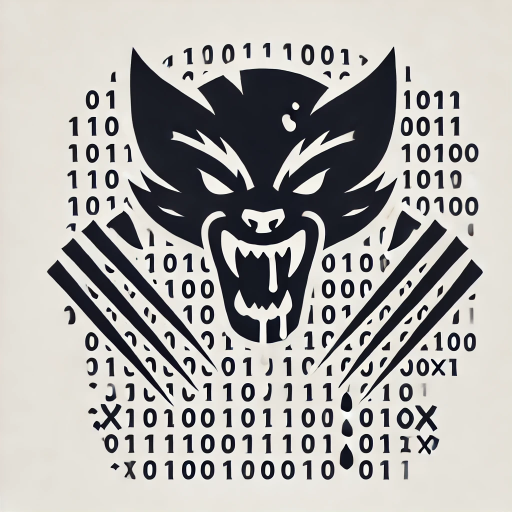
The challenge
A malware was provided from a real DFIR case that occurred in January 2024. The final payloads were disarmed here, to allow the analysts to dissect the binary safely until they fully understand the execution chain of a reflective shellcode loader named GuLoader [which initially led to the delivery of Remcos RAT with an additional keylogger, but the original nasty shellcodes were replaced by benign ones to provide the most realistic challenge].
Continue reading Insomni’hack 2025 – GuLosity writeupReinventing PowerShell in C/C++
I like PowerShell, I like it a lot! I like its versatility, its ease of use, its integration with the Windows operating system, but it also has a few features, such as AMSI, CLM, and other logging capabilities, that slow it down. You know, I’m thinking about the performance gain here. I believe my scripts could run a lot faster without them.
Jokes aside, I know that a lot has already been done around this subject, but I wanted to approach the problem in a slightly different way than the existing projects. So, I worked on a way to instantiate a full-blown PowerShell console using only native code, which allowed me to do some “cleaning” at the same time.
Continue reading Reinventing PowerShell in C/C++The effect of granting Azure Reader role on Azure Container Registry instances
We observed that granting Azure Reader role at subscription or resource group level allows users to pull container images from Azure Container Registry instances, thus potentially reveling confidential or sensitive data to unauthorised parties.
Continue reading The effect of granting Azure Reader role on Azure Container Registry instancesAttacking Cortex XDR from an unprivileged user perspective
In late 2023, we launched a new form of service where multiple customers could co-fund research time on a given product they are all using. The goal of the Co-funded research is to find vulnerabilities and possible weaknesses within the product that could impact not only our customers’ security, but anyone using the product. The discovered vulnerabilities are then reported to the editor of the solution and temporary mitigation options or IOCs are provided to the customers’ who funded the research.
In this context, we put some effort into the analysis of Cortex XDR and identified some interesting findings.
This blog post details two vulnerabilities (CVE-2024-5907 and CVE-2024-9469) that have now been fixed by Palo Alto and which could at the time be exploited by a low privileged user.
This research on Cortex XDR was performed by Florian Audon (@Nodauf) and Romain Melchiorre (@PMa1n).
Continue reading Attacking Cortex XDR from an unprivileged user perspectiveArbitrary web root file read in Sitecore before v10.4.0 rev. 010422
As part of our continuous pentesting offering, we try to identify solutions used by multiple clients to guide our research efforts to deliver the greatest impact. That is why, recently, we spent some time searching for vulnerabilities within Sitecore to find what we initially thought to be a 0-day, but ended up having been already patched some time earlier.
Continue reading Arbitrary web root file read in Sitecore before v10.4.0 rev. 010422Exploiting KsecDD through Server Silos
Earlier this year, an intriguing admin-to-kernel technique was published by @floesen_ in the form of a proof-of-concept (PoC) on GitHub. The author mentioned a strong limitation involving LSASS and Server Silos, without providing much details about it. This piqued our interest, so we decided to give it a second look…
Continue reading Exploiting KsecDD through Server SilosPrivilege escalation through TPM Sniffing when BitLocker PIN is enabled
This blog post offers additional insights following the presentation delivered at the Swiss Cyber Storm conference in Bern on October 22, 2024.
Continue reading Privilege escalation through TPM Sniffing when BitLocker PIN is enabledGetting code execution on Veeam through CVE-2023-27532
While several blog posts have shown how to retrieve credentials through this vulnerability, we decided to dig deeper and see whether it was possible to execute arbitrary code through this issue.
Continue reading Getting code execution on Veeam through CVE-2023-27532Ghost in the PPL Part 3: LSASS Memory Dump
Following my failed attempt to achieve arbitrary code execution within a protected LSASS process using the BYOVDLL technique and an N-day exploit in the KeyIso service, I took a step back, and reconsidered my life choices opted for a less ambitious solution: a (not so) simple memory dump. After all, when it comes to LSASS, we are mostly interested in extracting credentials stored in memory.